3. Python Growth Stock Screener
Welcome back to our Python for Finance series! Having explored value stocks and dividend payers, we now turn our attention to another exciting category: growth stocks. This post will show you how to build a Python growth stock screener to identify companies exhibiting strong expansion potential using yfinance
and pandas
.
What is a Growth Stock?
Growth investing focuses on companies expected to grow their revenue and earnings at an above-average rate compared to their industry or the overall market. Investors buy these stocks anticipating that their rapid expansion will lead to significant capital appreciation (stock price increase), often prioritizing growth potential over current profitability or dividend payouts.
Key characteristics often associated with growth stocks include:
- Strong Revenue Growth: The company is consistently increasing its sales.
- Rising Earnings Per Share (EPS): Profits attributable to each share are growing, indicating increasing profitability or efficiency.
- Innovation & Market Leadership: Often found in expanding industries or possessing competitive advantages that fuel growth.
Our goal is to use Python to filter stocks based on quantitative growth metrics.
Our Growth Screener Logic: Revenue and Earnings Momentum
For this screener, we’ll target companies showing recent strong performance in both top-line (revenue) and bottom-line (earnings) growth. We’ll use the following criteria, based on data potentially available in the yfinance
info
dictionary:
- Revenue Growth (> 10%): We’ll look for companies whose revenue has recently grown by more than 10%. The
yfinance
library provides arevenueGrowth
key. - Earnings Growth (> 10%): Similarly, we’ll screen for companies whose earnings have grown by more than 10%.
yfinance
offer anearningsGrowth
key (again, often quarterly year-over-year).
Important Note on Data: The specific growth metrics like revenueGrowth
and earningsGrowth
within yfinance
‘s info
dictionary can sometimes be inconsistent, unavailable for certain stocks, or represent different time periods (e.g., trailing twelve months vs. most recent quarter). This screener relies on their availability.
Our aim is to find companies demonstrating recent, significant expansion in both sales and profits, suggesting strong business momentum.
Python Implementation: Code for Screening Growth Stocks
Let’s write the Python script to identify stocks with high revenue and earnings growth. Make sure pandas
and yfinance
are installed (pip install pandas yfinance
).
- Imports & Setup: Import
pandas
,yfinance
, and manage warnings.
screen_growth_stocks
Function: Defines the main logic for our growth screener.
- Tickers & Storage: We define our
tickers
list and initializefiltered_stocks
.
- Looping & Fetching: Iterate through tickers, create
yf.Ticker
objects, and fetch theinfo
dictionary.
- Accessing Growth Metrics Safely: We use
info.get('revenueGrowth')
andinfo.get('earningsGrowth')
to retrieve the values. Using.get()
preventsKeyError
if these specific keys are missing for a stock, returningNone
instead. We store the company name usinginfo.get('shortName', 'N/A')
.
- Applying Growth Filters: We define our minimum growth thresholds (
min_growth_rate = 0.10
for 10%). The coreif
condition checks:- That both
revenue_growth
andearnings_growth
are notNone
(data is available). - That
revenue_growth
is greater thanmin_growth_rate
. - That
earnings_growth
is greater thanmin_growth_rate
.
- That both
- Storing Results: If a stock passes, its Ticker, Name, Revenue Growth, and Earnings Growth are added to the
filtered_stocks
list, formatted as percentages.
- DataFrame Creation & Output: The list is converted to a
pandas
DataFrame, and the results (or a message if none pass) are printed.
Python output High Growth Screener
Below is the output I get when running the code:
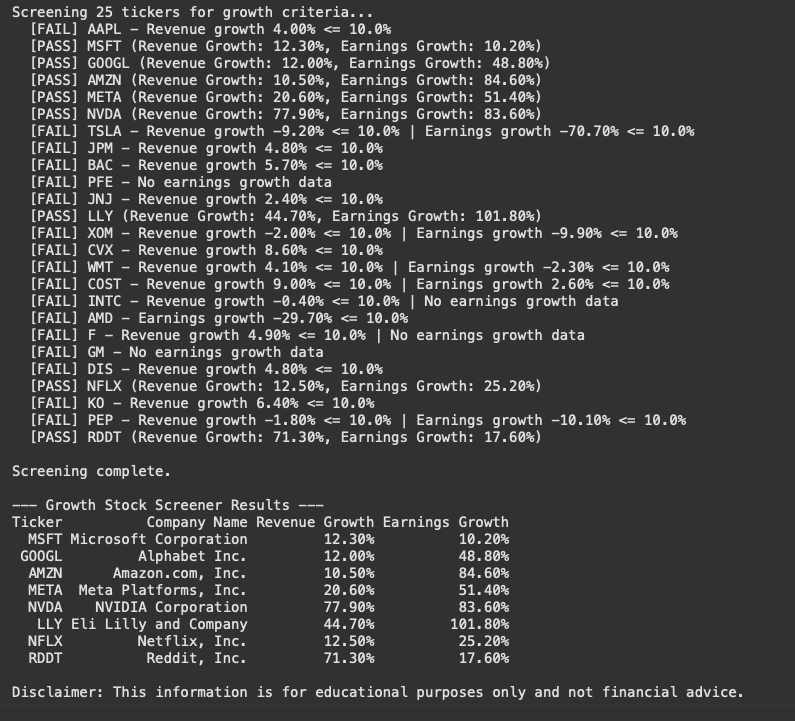
Companies like Microsoft, Alphabet (Google), Amazon, Meta, NVIDIA, Eli Lilly, Netflix, and Reddit all showed recent revenue and earnings growth rates exceeding the 10% threshold set in the Python script. They passed the growth screen.
Remember, this is based on potentially backward-looking data available at a specific moment and doesn’t guarantee future performance or consider valuation.
Also, always do a spot check when running Python code to ensure that you are retrieving the right data. For example, in Yahoo finance, in the statistics section, we can see the same Revenue and earnings growth as the code returned:
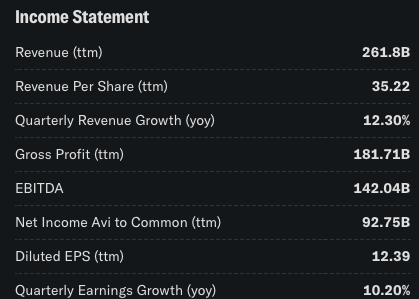
Limitations and Next Steps
This growth screener is a useful tool but has limitations:
- Data Dependency: Its effectiveness hinges on the availability and definition of the
revenueGrowth
andearningsGrowth
fields inyfinance
. These might not always be present or comparable across all stocks. - Backward-Looking: Like our previous screeners, it relies on past performance, which doesn’t guarantee future results. High growth can be difficult to sustain.
- Valuation Ignored: This screener doesn’t consider price. A high-growth stock might already be very expensive (high P/E ratio).
- No Qualitative Factors: It doesn’t account for management quality, competitive landscape, or industry trends.
Further research is essential. Investigate why the company is growing and whether that growth is sustainable and reasonably priced.
In our final post, we’ll explore combining screening factors (like value and growth for a GARP approach) and potentially adding simple technical indicators to refine our Python stock screeners.
Disclaimer: This educational content is not financial advice. Stock screening is only one part of investment analysis. Always conduct thorough due diligence or consult a qualified financial advisor before investing.