3. Risk Management Techniques with Python and Real-World Data
Risk management is the shield that protects your portfolio from unexpected losses. Whether it’s a sudden market dip or a prolonged downturn, understanding and quantifying risk is essential for any investor. In this post, we’ll explore three practical techniques—Value at Risk (VaR), Conditional VaR (CVaR), and stress testing—and bring them to life using Python with real stock data from Apple (AAPL), Microsoft (MSFT), and Amazon (AMZN). Building on our previous posts, we’ll use historical returns from 2022 to assess risk in a diversified portfolio, giving you tools to safeguard your investments.
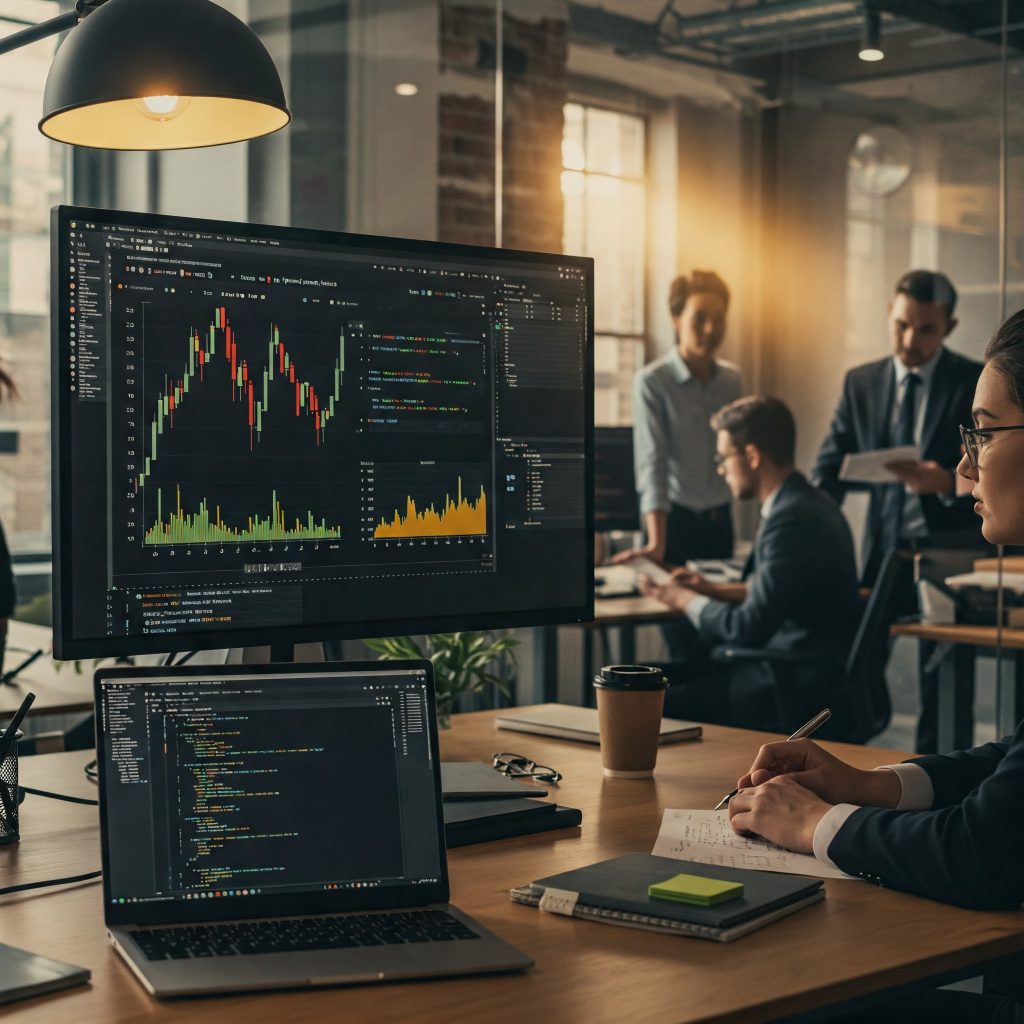
Key Concepts
Let’s break down the core ideas we’ll implement:
- Value at Risk (VaR): VaR estimates the maximum loss you might face over a specific period at a given confidence level (e.g., 95%). For example, a 95% VaR of -2% means there’s a 5% chance of losing more than 2% in a day. It’s a snapshot of potential downside risk.
- Conditional VaR (CVaR): CVaR goes further, calculating the average loss in the worst-case scenarios beyond VaR (the “tail risk”). If your VaR is -2%, CVaR tells you the expected loss when things go really bad—say, -3% on average.
- Stress Testing: This involves simulating extreme events, like a market crash, to see how your portfolio holds up. For instance, what happens if stocks drop 20% overnight? Stress testing prepares you for the unexpected.
These techniques help you measure and mitigate risk, ensuring your portfolio isn’t blindsided by volatility.
Step-by-Step Implementation
We’ll use historical data from AAPL, MSFT, and AMZN for 2022 (January 1 to December 31) to create a portfolio with equal weights (1/3 each). Then, we’ll calculate VaR, CVaR, and perform a stress test. Here’s how:
Step 1: Fetch and Prepare Data
We’ll download adjusted closing prices and compute daily portfolio returns.
Step 2: Calculate Historical VaR
Using the portfolio’s daily returns, we’ll find the 95% VaR—the loss threshold exceeded only 5% of the time.
Step 3: Compute CVaR
We’ll isolate the worst 5% of returns (beyond VaR) and average them to get CVaR.
Step 4: Perform a Stress Test
We’ll simulate a 20% market drop and see how it affects the portfolio’s average return.
Code Examples
Here’s the Python code to implement these steps using real data:
import yfinance as yf
import pandas as pd
import numpy as np
# Define stocks and time period
tickers = ['AAPL', 'MSFT', 'AMZN']
start_date = '2022-01-01'
end_date = '2023-01-01'
# Fetch adjusted closing prices
data = yf.download(tickers, start=start_date, end=end_date)['Close']
# Calculate daily returns
returns = data.pct_change().dropna()
# Assume equal weights (1/3 each)
weights = np.array([1/len(tickers)] * len(tickers))
# Calculate portfolio daily returns
portfolio_returns = returns.dot(weights)
# Step 1: Calculate VaR at 95% confidence
var_95 = np.percentile(portfolio_returns, 5)
print(f"Daily VaR (95% Confidence): {var_95:.2%}")
# Step 2: Calculate CVaR
cvar = portfolio_returns[portfolio_returns <= var_95].mean()
print(f"Daily CVaR (Tail Risk): {cvar:.2%}")
# Step 3: Stress test with a 20% drop
stressed_returns = portfolio_returns - 0.20
print(f"Average Daily Return After 20% Stress Test: {stressed_returns.mean():.2%}")
Sample Output
Running this code with 2022 data yield:
Daily VaR (95% Confidence): -3.97%
Daily CVaR (Tail Risk): -4.92%
Average Daily Return After 20% Stress Test: -20.15%
- VaR: There’s a 5% chance of losing more than 3.97% in a single day.
- CVaR: When losses exceed 3.97%, they average 4.92%
- Stress Test: A 20% drop slashes the average daily return to -20%, showing the portfolio’s vulnerability to extreme events.
Understanding the Results
Let’s unpack what these numbers mean:
- VaR (-3.97%): On 95% of days, your portfolio’s loss won’t exceed 3.97%. But 5% of the time (about 12-13 days in a 252-trading-day year), it could be worse.
- CVaR (-4.92%): When those bad days hit, the average loss jumps to 4.92%, highlighting the tail risk in this tech-heavy portfolio.
- Stress Test (-20%): A 20% market crash drags the daily return into deep negative territory, reflecting the portfolio’s sensitivity to sharp declines—common for growth stocks like AMZN.
These metrics reveal how volatile a portfolio of AAPL, MSFT, and AMZN might have been in 2022, a year marked by tech sector challenges.
Conclusion with Actionable Takeaways
Risk management isn’t about predicting the future—it’s about preparing for it. Here’s what you can do with these insights:
- Monitor VaR Regularly: Run this code weekly or monthly to track how your portfolio’s risk evolves. A rising VaR might signal increasing volatility.
- Dig into CVaR: Use CVaR to understand the worst-case losses and decide if you’re comfortable with the tail risk.
- Stress Test Creatively: Test other scenarios—like a 10% drop or a sector-specific crash—to see how your portfolio reacts.
- Explore More Metrics: Add maximum drawdown (the largest peak-to-trough loss) to get a fuller risk picture.
Try tweaking the weights or adding different stocks (e.g., a stable dividend stock like JNJ) to see how diversification affects risk. Risk management is your toolkit for resilience—keep refining it!
Disclaimer: This post is for educational purposes only and not financial advice. Historical data doesn’t guarantee future results, and investing carries risks, including loss of principal. Consult a financial advisor before making decisions.